What is good code
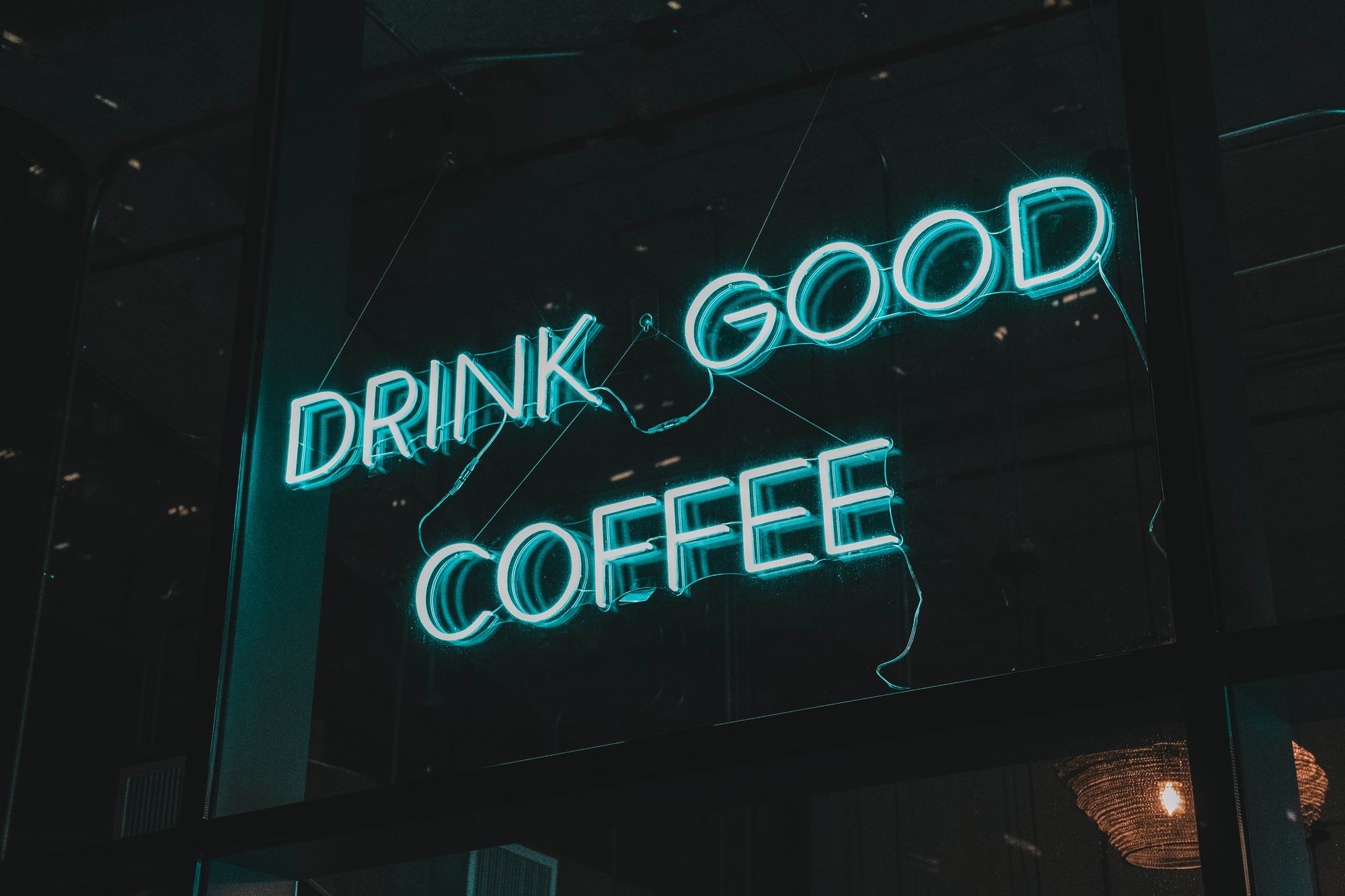
In the 2nd episode of Becoming a Senior Developer series, I'm talking about the definition of good code. What is good code? Is good code the same as correct code? When is it important to care about the code quality? These are some of the questions I answer in this video.
š Resources:
- https://en.wikipedia.org/wiki/ISO/IEC_9126 - ISO/IEC standard describing code quality
- https://www.researchgate.net/publication/227074069_Standardized_code_quality_benchmarking_for_improving_software_maintainability - paper describing code quality benchmarking
My notes from this episode:
Introduction
- today I'm going to talk about good code and whether good code matters
- the content I have was very long, more than 30 minutes
- so I split it into a few parts
- today I'm going to talk about characteristics of good code on a more abstract level
- in a few weeks I'm going to talk about a micro perspective, where I'll show you how to improve single functions and classes
- and we'll see where it goes from there
Characteristics of good code
Correct (functional)
- code can't be good if it's not correct
- correctness is not a binary concept
- code can work correctly in 50% of cases, 80% cases, 99.999% of cases
- you can write good code that is not 100% correct, if you're aware of the trade-offs you're making
Fast (efficient)
- good code is fast
- again, speed is not a binary concept
- depending on the type of the application, your code might produce outcome in 1ms, 1 minute or 1 day (provide examples)
- so by fast code I mean code that doesn't do unnecessary costly operations
- again, this is very relative and there might be a lot of discussions about it
- Examples:
- Code that does costly operation multiple times (solution: cache, memorization)
- Code that triggers N+1 db queries
- Code that uses suboptimal data structure (e.g. uses array instead of dictionary)
Secure
- good code is secure
- again, due to complexity of hardware and software we can't prevent 100% of security incidents
- so good code does not introduce new vulnerabilities to the application
Well designed (designed with change in mind)
- this is a section that I will talk more in the next videos
- the topic of maintainability is extremely broad
- today I will briefly mention a few characteristics of a well designed code
Easy to understand
- you can't change what you can't understand
- use descriptive variable names (don't ever call variables "data"!)
- use descriptive names for functions and classes
- keep functions and classes small and keep list of parameters short
- add comments to explain why certain code works the way it works
Easy to navigate
- you can't change what you can't find
- reduce redundancy - extract common parts
- split your code into classes and modules, keep related functions together
- ensure that different layers of the code do not mix, e.g. UI parts should not mix with business logic, business logic should not deal with data layer
Easy to test
- you can't change what you can't validate
- make sure to write automated tests wherever possible
- add a test for every bug that you find